Part 1:For the interactive Bezier Curve program, check the previous post.
Instead of showing a picture of my first program that drew triangles without clipping, here is a picture of the nearly finished program which first drew the complete triangles in green, then drew the clipped sections in red. I used this for debugging.
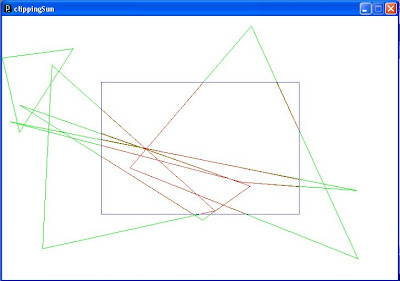
Here is a pic of the final version with fully implemented Cohen-Sutherland clipping:
Here is the code:
int x1, x2, x3, y1, y2, y3;
int xmax=(720/4)*3;
int xmin=720/4;
int ymax=(480/4)*3;
int ymin=480/4;
void draw(){
}
void setup() {
size(720, 480);
background(255,255,255);
stroke(0,0,255);
rect(xmin, ymin, 360,240);
stroke(0,0,0);
fiveTriangles();
}
void fiveTriangles(){
for(int i=0; i<5; i++){
x1=int(random(720));
x2=int(random(720));
x3=int(random(720));
y1=int(random(480));
y2=int(random(480));
y3=int(random(480));
Point p1=new Point(x1, y1);
Point p2=new Point(x2 ,y2);
Point p3=new Point(x3 ,y3);
myTri(p1, p2,p3);
}
}
void myTri(Point a, Point b, Point c){
stroke(0,255,0);
line(a.x,a.y,b.x,b.y);
line(b.x,b.y,c.x,c.y);
line(c.x,c.y,a.x,a.y);
stroke(255,0,0);
myLine(a,b);
myLine(b,c);
myLine(c,a);
}
void myLine(Point p1, Point p2){
int pCode=verify(p1);
int qCode=verify(p2);
if( (pCode & qCode)!=0 ) {
println("1) outside screen DONT DRAW");
}
else if( (pCode | qCode) == 0 )
{
line(p1.x, p1.y, p2.x, p2.y);
println("2) inside screen DRAW IT");
}
else
{
println("3) Gotta CLIP IT....");
clipLine(p1,p2);
}
}
void clipLine(Point P0, Point P1){
int outCode0=verify(P0);
int outCode1=verify(P1);
int x=P0.x;
int y=P0.y;
int x0=P1.x;
int y0=P1.y;
while((outCode0 | outCode1)!=0)
{
println("outCode0 | outCode1 = "+(outCode0 | outCode1)+"while loop");
//outCode0 = verify(P0);
//outCode1 = verify(P1);
if(outCode0 == 0)
{
println("switching P and Q");
Point tempPt; int tempCode;
tempPt = P0;
P0= P1;
P1 = tempPt;
tempCode = outCode0;
outCode0 = outCode1;
outCode1 = tempCode;
tempCode=x;
x=x0;
x0=tempCode;
tempCode=y;
y=y0;
y0=tempCode;
}
if( (outCode0 & 1) != 0 )
{
println("outCode0 & 1 = "+(outCode0 & 1)+"clip at BOTTOM");
x = P0.x + (P1.x - P0.x)*(ymax - P0.y)/(P1.y - P0.y);
y = ymax;
outCode0=verify(x,y);
}
else
if( (outCode0 & 2) != 0 )
{
println("outCode0 & 2 = "+(outCode0 & 2)+"clip at TOP");
x=P0.x + (P1.x - P0.x)*(ymin - P0.y)/(P1.y - P0.y);
y = ymin;
outCode0=verify(x,y);
}
else
if( (outCode0 & 4) != 0 )
{
println("outCode0 & 4 = "+(outCode0 & 4)+"clip at RIGHT");
y =P0.y + (P1.y - P0.y)*(xmax - P0.x)/(P1.x - P0.x);
x = xmax;
outCode0=verify(x,y);
}
else
if( (outCode0 & 8) != 0 )
{
println("outCode0 & 8 = "+(outCode0 & 8)+"clip at LEFT");
y = P0.y + (P1.y - P0.y)*(xmin - P0.x)/(P1.x - P0.x);
x = xmin;
outCode0=verify(x,y);
}
//outCode0 = verify(P0);
//outCode1 = verify(P1);
}
println("FINALLY clipping done, drawing line!!!");
line(x, y, x0, y0);
}
int verify(Point p)
{
int bits=0;
if(p.x bits +=8;
if(p.x>xmax)
bits +=4;
if(p.y bits +=2;
if(p.y>ymax)
bits +=1;
String s=binary(bits,4);
println(s);
return bits;
}
int verify(int x, int y)
{
int bits=0;
if(x bits +=8;
if(x>xmax)
bits +=4;
if(y bits +=2;
if(y>ymax)
bits +=1;
String s=binary(bits,4);
println(s);
return bits;
}
Part 2
For my project
I propose a program that will create creatures that have a recognizable structure, but are also created using some random values so that no two would be alike. This concept could be taken further by making the program interactive, so that if you click on two of the creatures you would get a third creature that "inherits" characteristics of both. The creatures would be drawn using primitive lines, circles, curves etc but would be detailed enough to look good. Here is a picture of a NodeBox project called Aquatics which inspired this idea. I would want to implement it in Processing rather than NodeBox because I don't have a Mac. Click on the link to view the original project page.